In this article, I’ll show you how to create pagination with node js , MongoDB, Express.js, EJS, and Bootstrap. We will generate a new database, define data collection, fill this collection with information and display contents to the page using pagination.
Suppose we have a database that includes a lot of information (e.g. items) and we want to see everything on the website. We can only output items on the page, but it doesn’t look so good. We need pagination in such cases to display the results as a good format.
This post is generally designed for beginners who are involved in web development, so I will try to create all the explanations very detailed. But maybe this article will be helpful to an experienced developer.
Before start, you need to have Node.js, express.js, and MongoDB installed to read this post. You can download it from official websites and install it:
Node.js – https://nodejs.org/en/
See: How to Setup Environment to run node js code
See: How to install express
See: How to use mongoose with node.js
Get started by creating a fresh folder for this task and name it whatever you like. Then create additional folders and files within that directory to match the following structure:
2 3 4 5 6 7 8 9 10 11 12 |
. ├── routes | └── index.js ├── models | └── add_password.js ├── views ├── add-new-password.ejs | └── view-all-password.ejs |
Open models/add_password.js
file and copy and paste the following code to create schema and mongoose connection:
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
const mongoose = require('mongoose'); mongoose.connect('mongodb://localhost:27017/pms', {useNewUrlParser: true, useCreateIndex: true,}); var conn =mongoose.Collection; var passSchema =new mongoose.Schema({ password_category: {type:String, required: true, index: { unique: true, }}, project_name: {type:String, required: true, }, password_detail: {type:String, required: true, }, date:{ type: Date, default: Date.now } }); var passModel = mongoose.model('password_details', passSchema); module.exports=passModel; |
In this file we create a connection to MongoDB, PMS is a database name and password_details is a collection name.
Open routes/index.js
file and copy and paste the following code to create POST route and a new page with HTML form :
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
var express = require('express'); var router = express.Router(); var passModel = require('../modules/add_password'); router.get('/add-new-password',function(req, res, next) { res.render('add-new-password'); }); router.post('/add-new-password',function(req, res, next) { var pass_cat= req.body.pass_cat; var project_name= req.body.project_name; var pass_details= req.body.pass_details; var password_details= new passModel({ password_category:pass_cat, project_name:project_name, password_detail:pass_details }); password_details.save(function(err,doc){ res.render('add-new-password', { success:"Password Details Inserted Successfully"}); }); }); |
Let’s create views/add-new-password.ejs
page with form to send a POST
request to our POST
route.
Open views/add-new-password.ejs
file and paste following HTML:
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 |
<!DOCTYPE html> <html> <head> <title>Password Management System</title> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.4.0/css/font-awesome.min.css"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.0/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/js/bootstrap.min.js"></script> <script src="https://cdn.ckeditor.com/4.9.1/standard/ckeditor.js"></script> </head> <body> <nav class="navbar navbar-default"> <div class="container"> <div class="navbar-header"> <a class="navbar-brand" href="#">Password Management System</a> </div> <ul class="nav navbar-nav"> <li class="active"><a href="/">Home</a></li> <li class="dropdown"><a href="#" class="dropdown-toggle" data-toggle="dropdown">Password Category </a> <ul class="dropdown-menu"> <li class="dropdown"><a href="#" class="dropdown-toggle" data-toggle="dropdown">Password Details </a> <ul class="dropdown-menu"> <li><a href="add-new-password" class="dropdown-item">Add New Password</a></li> <li><a href="view-all-password" class="dropdown-item">View All Password</a></li> </ul></li> </ul> </div> </nav> <div class="container"> <div class="row"> <div id="signupbox" style="margin-top:50px" class="mainbox col-md-6 col-md-offset-3 col-sm-8 col-sm-offset-2"> <div class="panel panel-info"> <div class="panel-heading"> <div class="panel-title"> Add New Password</div> </div> <div class="panel-body" > <form action="/add-new-password" id="passwordform" class="form-horizontal" method="post" role="form"> <div class="form-group"> <label for="username" class="col-md-3 control-label">Password Details</label> <div class="col-md-9"> <select class="form-control" name="pass_cat"> <option value="">Choose Password Category</option> <option value="Godaddy">Godaddy</option> <option value="Cpanel">Cpanel</option> <option value="Gmail">Gmail</option> <option value="Yahoo">Yahoo</option> </select> </div> </div> <div class="form-group"> <label for="username" class="col-md-3 control-label">Project Name</label> <div class="col-md-9"> <input type="textbox" class="form-control" name="project_name" placeholder="Enter Your Project Name"> </div> </div> <div class="form-group"> <label for="username" class="col-md-3 control-label">Password Details</label> <div class="col-md-9"> <textarea class="form-control" id="editor" name="pass_details" rows="5" placeholder="Enter Your Password Details"></textarea> </div> </div> <div class="form-group"> <!-- Button --> <div class="col-md-offset-3 col-md-9"> <input type="submit" name="submit" value="Add Password" class="btn btn-primary"> </div> </div> <p><a href="/view-all-password">View All Password Lists</a></p> </form> </div> </div> </div> </div> </div> <script> CKEDITOR.replace( 'editor' ); </script> </body> </html> |
Now you can run your MongoDB, then run a server with node index.js
command and go to
http://localhost:3000/add-new-password
For easy viewing and editing of MongoDB content on your desktop, I suggested to use MongoDB Compass
Add a new Password Details:
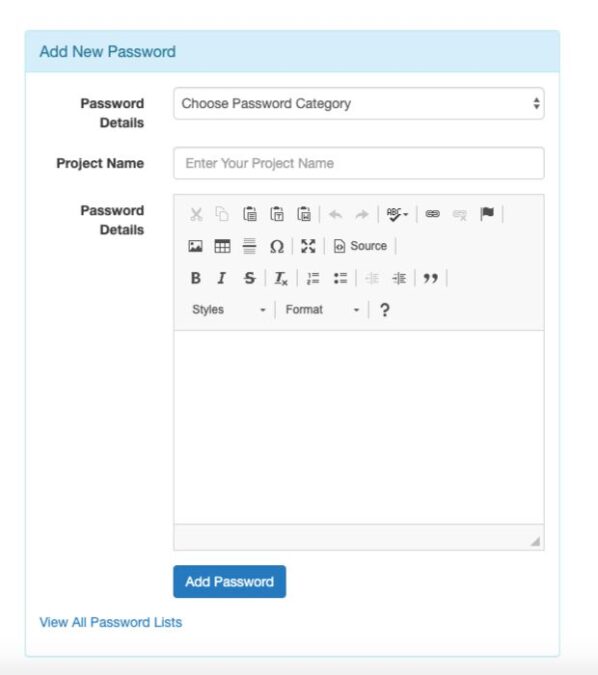
View in MongoDB Compass:
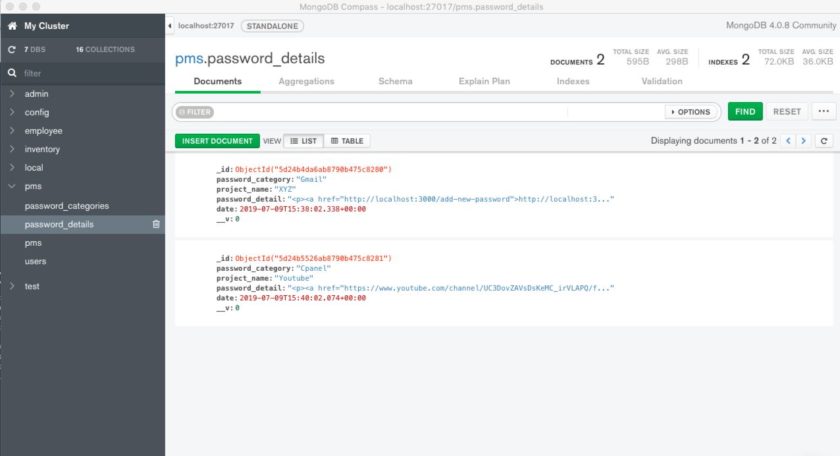
Output Password Details to Page with Pagination
First we need to create new route to render view-all-password
page and output some necessary data to create pagination. Open routes/index.js
file and paste following code:
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
router.get('/view-all-password/', function(req, res, next) { var perPage = 1; var page = req.params.page || 1; passModel.find({}) .skip((perPage * page) - perPage) .limit(perPage).exec(function(err,data){ if(err) throw err; passModel.countDocuments({}).exec((err,count)=>{ res.render('view-all-password', { records: data, current: page, pages: Math.ceil(count / perPage) }); }); }); }); router.get('/view-all-password/:page', function(req, res, next) { var perPage = 1; var page = req.params.page || 1; passModel.find({}) .skip((perPage * page) - perPage) .limit(perPage).exec(function(err,data){ if(err) throw err; passModel.countDocuments({}).exec((err,count)=>{ res.render('view-all-password', { records: data, current: page, pages: Math.ceil(count / perPage) }); }); }); }); |
Let me explain:
perPage
variable contains max number of items on each page, page
variable contains the current page number.
For each page, we need to skip ((perPage * page) – perPage) values (on the first page the value of the skip should be 0). output only perPage
items as a limit.
Count all items in the collection with count()
(we will use this value to calculate the number of pages
then render view-all-password
page and send necessary data.
Create pagination with node js page:
Open views/view-all-password.ejs
and paste following EJS:
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 |
<!DOCTYPE html> <html> <head> <title>Password Management System</title> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.4.0/css/font-awesome.min.css"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.0/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/js/bootstrap.min.js"></script> </head> <body> <nav class="navbar navbar-default"> <div class="container"> <div class="navbar-header"> <a class="navbar-brand" href="#">Password Management System</a> </div> <ul class="nav navbar-nav"> <li class="active"><a href="/">Home</a></li> <li class="dropdown"><a href="#" class="dropdown-toggle" data-toggle="dropdown">Password Details </a> <ul class="dropdown-menu"> <li><a href="add-new-password" class="dropdown-item">Add New Password</a></li> <li><a href="view-all-password" class="dropdown-item">View All Password</a></li> </ul></li> </ul> </nav> <div class="container"> <div class="row"> <h1>View Password Lists</h1> <br> <table class="table table-striped table-primary"> <thead> <tr> <th>Password Category Name</th> <th>Project Name</th> <th>Action</th> </tr> </thead> <tbody> <% if(records.length>0){ records.forEach(function(row){ %> <tr> <td><%= row.password_category %></td> <td><%= row.project_name %></td> <td> <button type="button" class="btn btn-info btn-lg" data-toggle="modal" data-target="#myModal<%= row._id %>"><i class="fa fa-eye"></i></button> </td> </tr> <!-- Modal --> <div class="modal fade" id="myModal<%= row._id %>" role="dialog"> <div class="modal-dialog"> <!-- Modal content--> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal">×</button> <h4 class="modal-title"><%= row.project_name %></h4> </div> <div class="modal-body"> <%- row.password_detail %> </div> <div class="modal-footer"> <button type="button" class="btn btn-default" data-dismiss="modal">Close</button> </div> </div> </div> </div> <% })} %> </tbody> </table> <% if (pages > 0) { %> <ul class="pagination text-center"> <% if (current == 1) { %> <li class="disabled"><a>First</a></li> <% } else { %> <li><a href="/view-all-password/1">First</a></li> <% } %> <% var i = (Number(current) > 5 ? Number(current) - 4 : 1) %> <% if (i !== 1) { %> <li class="disabled"><a>...</a></li> <% } %> <% for (; i <= (Number(current) + 4) && i <= pages; i++) { %> <% if (i == current) { %> <li class="active"><a><%= i %></a></li> <% } else { %> <li><a href="/view-all-password/<%= i %>"><%= i %></a></li> <% } %> <% if (i == Number(current) + 4 && i < pages) { %> <li class="disabled"><a>...</a></li> <% } %> <% } %> <% if (current == pages) { %> <li class="disabled"><a>Last</a></li> <% } else { %> <li><a href="/view-all-password/<%= pages %>">Last</a></li> <% } %> </ul> <% } %> </div> </div> </body> </html> |
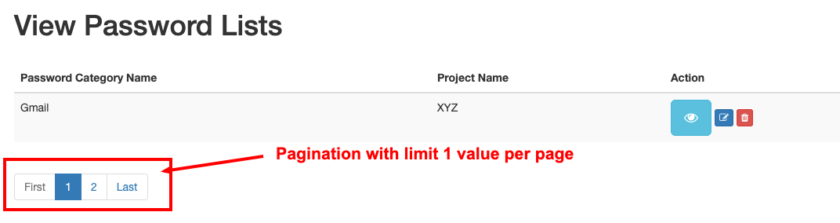
Let me explain:
you will show pagination only if the number of pages more than 0:
2 3 4 |
<% if (pages > 0) { %> |
2 3 4 |
<% var i = (Number(current) > 5 ? Number(current) - 4 : 1) %> |
Here check number of current page and if this value less than 5 we are output pagination links from 1 to current_page + 4
:
2 3 4 |
<% for (; i <= (Number(current) + 4) && i <= pages; i++) { %> |
and if this value more than 5 we will start output pagination link from current_page - 4
to current_page + 4
, thus previous links on current_step - 5
will be hide.
Save HTML Form Record into MongoDB Database Using Express js and Mongoose js
Render Mongodb Data Records in HTML using EJS View Engine and express js
Conclusion:
This is How to Create Pagination with Node js, MongoDB, Express and EJS. You can use and customize this code according to your requirements. Are you want to get implementation help, or modify or extend the functionality of this script? Submit paid service request
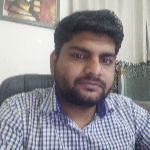
Pradeep Maurya is the Professional Web Developer & Designer and the Founder of “Tutorials website”. He lives in Delhi and loves to be a self-dependent person. As an owner, he is trying his best to improve this platform day by day. His passion, dedication and quick decision making ability to stand apart from others. He’s an avid blogger and writes on the publications like Dzone, e27.co